Introduction
Chatbots, which provide automated assist and individualised experiences, have revolutionised the best way that companies join with their clients. Current developments in synthetic intelligence (AI) have raised the bar for chatbot performance. In-depth directions on making a customized chatbot utilizing OpenAI, a number one AI platform famend for its potent language fashions, are offered on this detailed guide.
This text was printed as part of the Knowledge Science Blogathon.
What are Chatbots?
Chatbots are laptop programmes that mimic human conversations. They make use of pure language processing (NLP) strategies to understand what customers are saying and reply in a related and useful method.
Due to the supply of large datasets and glorious machine studying algorithms, chatbots have gotten more and more clever lately. These capabilities allow chatbots to raised grasp person intent and ship replies that sound extra real.
Some concrete situations of how chatbots at the moment are getting used:
- Chatbots in customer support could reply generally requested inquiries and provides assist to customers across the clock.
- Chatbots in advertising and marketing could help companies in qualifying leads, producing leads, and answering queries about services or products.
- Chatbots in schooling can provide personalised teaching and permit college students to check at their very own velocity.
- Chatbots in healthcare can provide details about well being considerations, reply drug inquiries, and hyperlink sufferers with physicians or different healthcare professionals.
Introduction to OpenAI
OpenAI is on the forefront of synthetic intelligence analysis and growth. It has led the best way within the creation of cutting-edge language fashions that excel at deciphering and creating pure language.
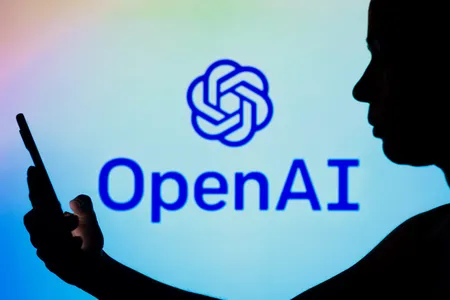
OpenAI gives refined language fashions similar to GPT-4, GPT-3, Textual content-davinci which is extensively used for NLP actions similar to chatbot constructing and plenty of extra.
Benefits of Utilizing Chatbots
Let’s first comprehend some advantages of utilizing chatbots earlier than entering into the coding and implementation:
- 24/7 Availability: Chatbots could supply customers round the clock help, eliminating the bounds of human customer support representatives and permitting companies to fulfill their shoppers’ calls for every time they come up.
- Improved Buyer Service: Chatbots could reply to regularly requested inquiries in a well timed method by offering correct and speedy solutions. This improves the general high quality of consumer service.
- Price Financial savings: Corporations can save some huge cash over time by automating buyer assist chores and decreasing the necessity for a giant assist workers.
- Elevated Effectivity: Chatbots can handle a number of conversations without delay, guaranteeing speedy responses and reducing down on person wait occasions.
- Knowledge Assortment and Evaluation: Chatbots could collect helpful info from person interactions, giving firms an understanding of consumer preferences, needs, and ache spots. Utilizing this knowledge, enhance the services.
Let’s go on to the step-by-step breakdown of the code wanted to construct a bespoke chatbot utilizing OpenAI now that we’re conscious of some great benefits of chatbots.
Steps
Step 1: Importing the Required Libraries
We have to import the mandatory libraries. Within the offered code, we will see the next import statements:
!pip set up langchain
!pip set up faiss-cpu
!pip set up openai
!pip set up llama_index
# or you need to use
%pip set up langchain
%pip set up faiss-cpu
%pip set up openai
%pip set up llama_index
Be sure you have these libraries put in earlier than shifting on.
Step 2: Setting Up the API Key
To work together with the OpenAI API, you want an API key. Within the offered code, there’s a placeholder indicating the place so as to add your API key:
To seek out you API Key, go to openai web site and create a brand new open ai key.
import os
os.environ["OPENAI_API_KEY"] = 'Add your API Key right here'
Change ‘Add your API Key right here’ along with your precise API key obtained from OpenAI.
Step 3: Creating and Indexing the Information Base
On this step, we’ll create and index the information base that the chatbot will discuss with for answering person queries. The offered code demonstrates two approaches: one for loading paperwork from a listing and one other for loading an present index. Let’s deal with the primary strategy.
from llama_index import GPTVectorStoreIndex, SimpleDirectoryReader
paperwork = SimpleDirectoryReader('/Customers/tarakram/Paperwork/Chatbot/knowledge').load_data()
print(paperwork)
Use the SimpleDirectoryReader class to load the paperwork from a particular listing.
Change ‘/Customers/tarakram/Paperwork/Chatbot/knowledge’ with the trail to your listing containing the information base paperwork. The load_data() operate masses the paperwork and returns them.
After loading the paperwork, we have to create an index utilizing the GPTVectorStoreIndex class:
index = GPTVectorStoreIndex.from_documents(paperwork)
This step creates the index utilizing the loaded paperwork.
Step 4: Persisting the Index
To keep away from the necessity for rebuilding the index each time the code runs, we will persist the index to disk. Within the offered code, the next line is used to save lots of the index:
# Save the index
index.storage_context.persist('/Customers/tarakram/Paperwork/Chatbot')
Make certain to switch ‘/Customers/tarakram/Paperwork/Chatbot’ with the specified listing path the place you need to save the index.
By persisting the index, we will load it in subsequent runs with out incurring extra token prices.
Step 5: Loading the Index
In case you need to load the beforehand saved index, you need to use the next code:
from llama_index import StorageContext, load_index_from_storage
# rebuild storage context
storage_context = StorageContext.from_defaults
(persist_dir="/Customers/tarakram/Paperwork/Chatbot/index")
# load index
index = load_index_from_storage(storage_context)
Make sure that you replace ‘/Customers/tarakram/Paperwork/Chatbot/index’ with the proper listing path the place you save the index.
Step 6: Creating the Chatbot Class
Now, let’s transfer on to creating the precise chatbot class that interacts with the person and generates responses. Right here’s the offered code:
# Chat Bot
import openai
import json
class Chatbot:
def __init__(self, api_key, index):
self.index = index
openai.api_key = api_key
self.chat_history = []
def generate_response(self, user_input):
immediate = "n".be a part of([f"{message['role']}: {message['content']}"
for message in self.chat_history[-5:]])
immediate += f"nUser: {user_input}"
query_engine = index.as_query_engine()
response = query_engine.question(user_input)
message = {"position": "assistant", "content material": response.response}
self.chat_history.append({"position": "person", "content material": user_input})
self.chat_history.append(message)
return message
def load_chat_history(self, filename):
attempt:
with open(filename, 'r') as f:
self.chat_history = json.load(f)
besides FileNotFoundError:
move
def save_chat_history(self, filename):
with open(filename, 'w') as f:
json.dump(self.chat_history, f)
The Chatbot class has an __init__ technique to initialize the chatbot occasion with the offered API key and index.
The generate_response technique takes person enter, generates a response utilizing the index and OpenAI API, and updates the chat historical past.
The load_chat_history and save_chat_history strategies are used to load and save the chat historical past, respectively.
Step 7: Interacting with the Chatbot
The ultimate step is to work together with the chatbot. Right here’s the offered code snippet that demonstrates find out how to use the chatbot:
bot = Chatbot("Add your API Key right here ", index=index)
bot.load_chat_history("chat_history.json")
whereas True:
user_input = enter("You: ")
if user_input.decrease() in ["bye", "goodbye"]:
print("Bot: Goodbye!")
bot.save_chat_history("chat_history.json")
break
response = bot.generate_response(user_input)
print(f"Bot: {response['content']}")
To make use of the chatbot, create an occasion of the Chatbot class by passing your OpenAI API key and the loaded index.
Change “Add your API Key right here ” along with your precise API key. The load_chat_history technique is used to load the chat historical past from a file (change “chat_history.json” with the precise file path).
Then, some time loop is used to repeatedly get person enter and generate responses till the person enters “bye” or “goodbye.”
The save_chat_history technique is used to save lots of the chat historical past to a file.
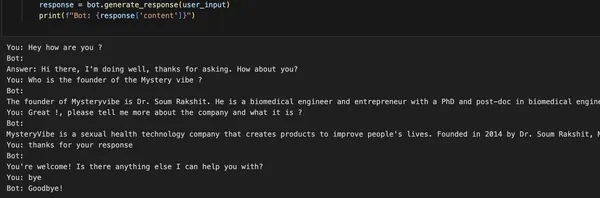
Step 8: Constructing a Internet Software utilizing Streamlit
The code offered additionally features a net utility constructed utilizing Streamlit, permitting customers to work together with the chatbot by way of a person interface. Right here’s the offered code:
import streamlit as st
import json
import os
from llama_index import StorageContext, load_index_from_storage
os.environ["OPENAI_API_KEY"] = 'Add your API Key right here '
# rebuild storage context
storage_context = StorageContext.from_defaults
(persist_dir="/Customers/tarakram/Paperwork/Chatbot/index")
# load index
index = load_index_from_storage(storage_context)
# Create the chatbot
# Chat Bot
import openai
import json
class Chatbot:
def __init__(self, api_key, index, user_id):
self.index = index
openai.api_key = api_key
self.user_id = user_id
self.chat_history = []
self.filename = f"{self.user_id}_chat_history.json"
def generate_response(self, user_input):
immediate = "n".be a part of([f"{message['role']}: {message['content']}"
for message in self.chat_history[-5:]])
immediate += f"nUser: {user_input}"
query_engine = index.as_query_engine()
response = query_engine.question(user_input)
message = {"position": "assistant", "content material": response.response}
self.chat_history.append({"position": "person", "content material": user_input})
self.chat_history.append(message)
return message
def load_chat_history(self):
attempt:
with open(self.filename, 'r') as f:
self.chat_history = json.load(f)
besides FileNotFoundError:
move
def save_chat_history(self):
with open(self.filename, 'w') as f:
json.dump(self.chat_history, f)
# Streamlit app
def foremost():
st.title("Chatbot")
# Consumer ID
user_id = st.text_input("Your Identify:")
# Test if person ID is offered
if user_id:
# Create chatbot occasion for the person
bot = Chatbot("Add your API Key right here ", index, user_id)
# Load chat historical past
bot.load_chat_history()
# Show chat historical past
for message in bot.chat_history[-6:]:
st.write(f"{message['role']}: {message['content']}")
# Consumer enter
user_input = st.text_input("Sort your questions right here :) - ")
# Generate response
if user_input:
if user_input.decrease() in ["bye", "goodbye"]:
bot_response = "Goodbye!"
else:
bot_response = bot.generate_response(user_input)
bot_response_content = bot_response['content']
st.write(f"{user_id}: {user_input}")
st.write(f"Bot: {bot_response_content}")
bot.save_chat_history()
bot.chat_history.append
({"position": "person", "content material": user_input})
bot.chat_history.append
({"position": "assistant", "content material": bot_response_content})
if __name__ == "__main__":
foremost()
To run the online utility, guarantee you could have Streamlit put in (pip set up streamlit).
Change “Add your API Key right here ” along with your precise OpenAI API key.
Then, you possibly can run the applying utilizing the command streamlit run app.py.
The online utility will open in your browser, and you may work together with the chatbot by way of the offered person interface.
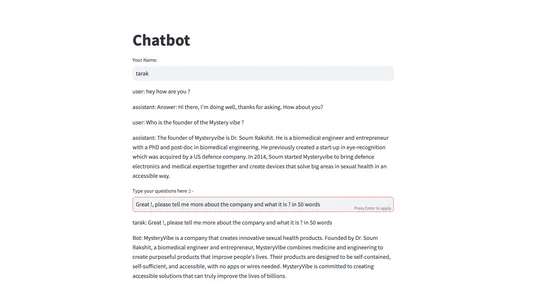
Bettering the Efficiency of Chatbots
Contemplate the next methods to enhance chatbot efficiency:
- High quality-tuning: Constantly improve the chatbot mannequin’s comprehension and response producing abilities by fine-tuning it with extra knowledge.
- Consumer Suggestions Integration: Combine person suggestions loops to gather insights and improve chatbot efficiency primarily based on real-world person interactions.
- Hybrid Methods: Examine hybrid strategies that mix rule-based programs with AI fashions to raised successfully deal with difficult circumstances.
- Area-Particular Data: Embrace domain-specific info and knowledge to extend the chatbot’s experience and accuracy in sure matter areas.
Conclusion
Congratulations! You’ve now discovered find out how to create a customized chatbot utilizing OpenAI. On this Information, we checked out find out how to use OpenAI to create a bespoke chatbot. We coated the steps concerned in establishing the required libraries, acquiring an API key, creating and indexing a information base, creating the chatbot class, and interacting with the chatbot.
You additionally explored the choice of constructing an online utility utilizing Streamlit for a user-friendly interface.Making a chatbot is an iterative course of, and fixed refinement is crucial for enhancing its performance. You’ll be able to design chatbots that give excellent person experiences and significant assist by harnessing the ability of OpenAI and staying updated on the newest breakthroughs in AI. Experiment with totally different prompts, coaching knowledge, and fine-tuning strategies to create a chatbot tailor-made to your particular wants. The chances are limitless, and OpenAI gives a robust platform to discover and unleash the potential of chatbot expertise.
Key Takeaways
- Establishing the mandatory libraries, acquiring an API key, producing and indexing a information base, and implementing the chatbot class are all steps within the means of developing a bespoke chatbot utilizing OpenAI.
- Chatbots are laptop programmes that mimic human interactions by offering assist and answering questions in pure language.
- Knowledge gathering is crucial for coaching an environment friendly chatbot, and it entails buying related and diverse datasets from reliable sources.
- Conversational AI purposes utilizing OpenAI’s GPT-3.5 language mannequin, a potent software for pure language processing.
- Utilizing instruments just like the Llamas library to index and search a information base can significantly enhance the chatbot’s capability to retrieve pertinent knowledge.
- Streamlit gives a sensible framework for creating net purposes that allow customers to speak with the chatbot through an intuitive person interface.
You’ll be able to entry the code in Github – Hyperlink
Join with me in Linkedin – Hyperlink
Often Requested Questions
A. OpenAI created the potent language mannequin GPT-3.5. Primarily based on directions or conversations, it may well comprehend and produce textual content that appears and sounds human.
A. Create an account on the OpenAI web site and observe the on-screen directions to realize entry to the API with a purpose to acquire an API key for OpenAI.
A. Information base indexing is structuring and organising a physique of information in order that it may be discovered and retrieved rapidly. It’s essential for a chatbot because it allows the bot to acquire pertinent knowledge and provides exact solutions to person inquiries.
A. Sure, by including extra coaching knowledge and altering the prompts used to generate responses, you possibly can fine-tune the chatbot’s responses. By doing this, it’s possible you’ll help the chatbot turn into extra tailor-made to your focused use case.
The media proven on this article is just not owned by Analytics Vidhya and is used on the Writer’s discretion.